Crypto Sudoku Game Documentation
A comprehensive guide to the Web3-integrated Sudoku puzzle game
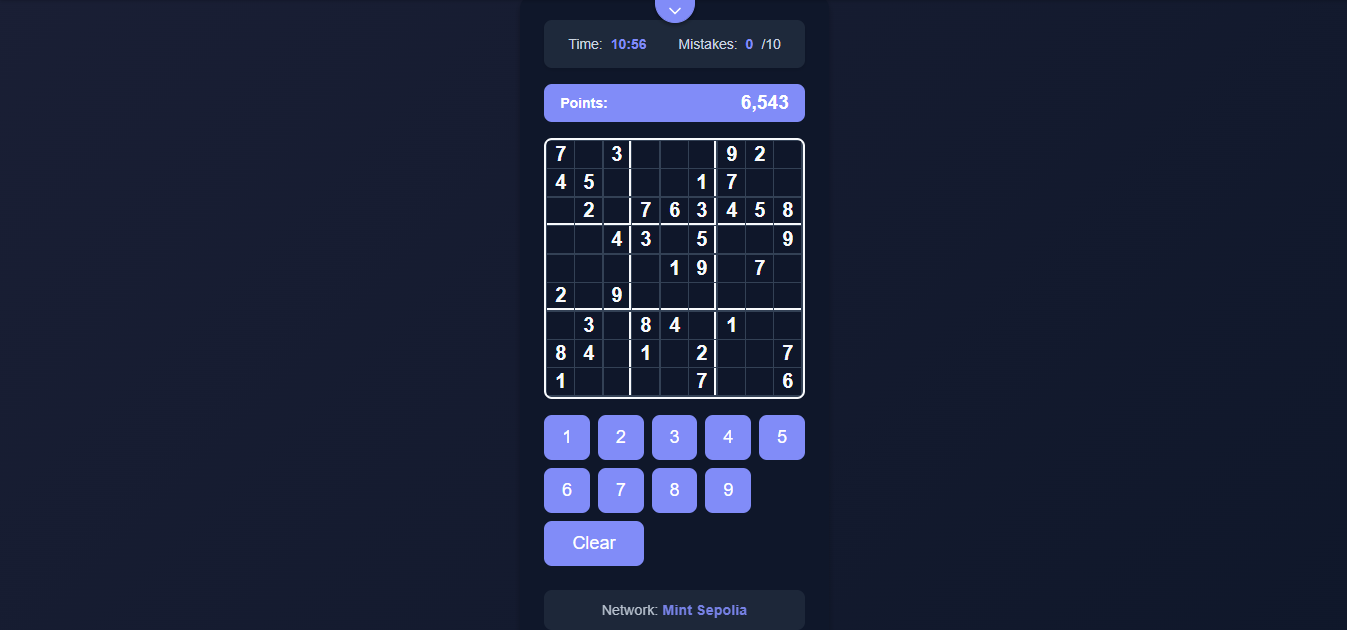
Overview
Classic Sudoku
Traditional Sudoku puzzle game with engaging gameplay.
NFT Gated
Access requires ownership of a Crypto Sudoku NFT on Mint Sepolia or Monad Testnet network.
Leaderboard
Submit your scores to a blockchain-based leaderboard system.
Dark Mode
Light and dark themes for comfortable gameplay in any setting.
Key Features
Auto-Generated Puzzles
Every game generates a unique puzzle with a guaranteed solution. Each puzzle is carefully designed for an enjoyable challenge.
NFT Authentication
Securely authenticate using your Web3 wallet. Access is granted only to users who own a Crypto Sudoku NFT, with a maximum supply of 10,000 NFTs.
Seasonal Leaderboards
Complete puzzles faster to climb the leaderboard. Each season offers a fresh start for competition.
Audio Feedback
Get satisfying audio feedback for correct and incorrect moves. Sound can be toggled on or off.
How to Play
Connect Your Wallet
Use the "Connect Wallet" button to authenticate with your Web3 wallet (MetaMask, WalletConnect, etc.).
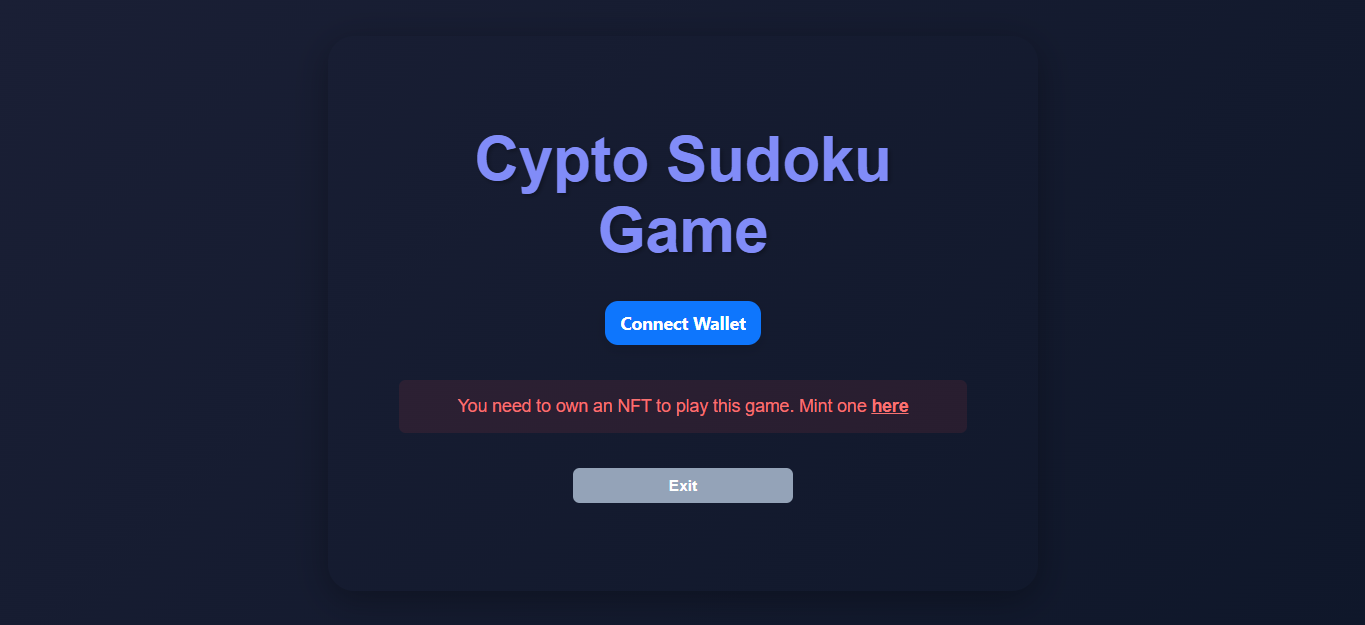
Minting NFTs
Mint your Crypto Sudoku NFT on your selected network to gain access to the game. Each wallet can mint only one NFT (except for the contract owner).
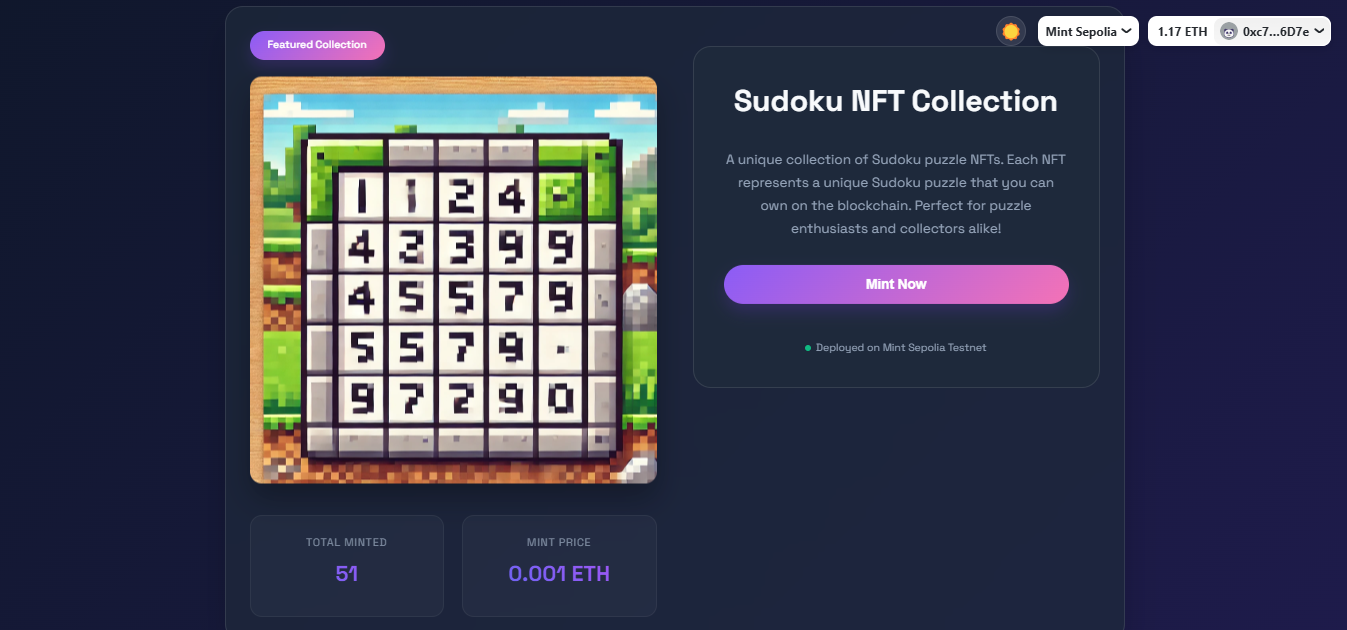
Choose Your Network
Select between Mint Sepolia or Monad Testnet networks. Each network has its own NFTs and leaderboard.
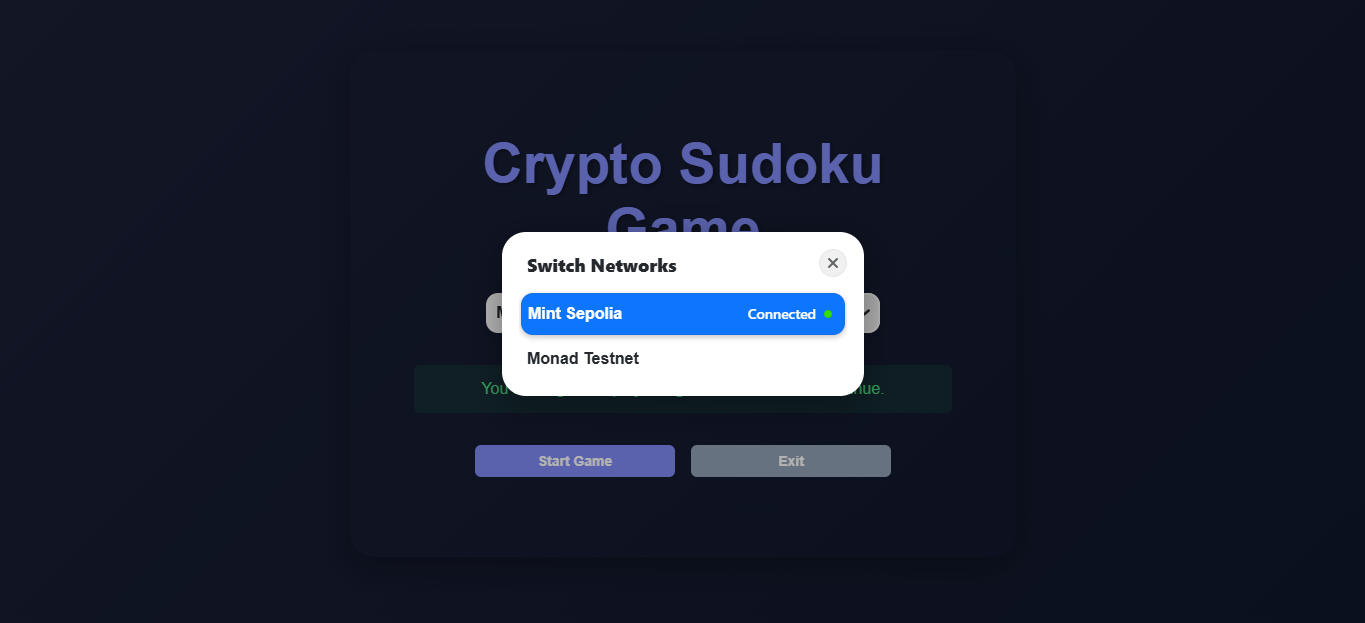
Start Game
Start the game if you already have the NFT on your selected network.
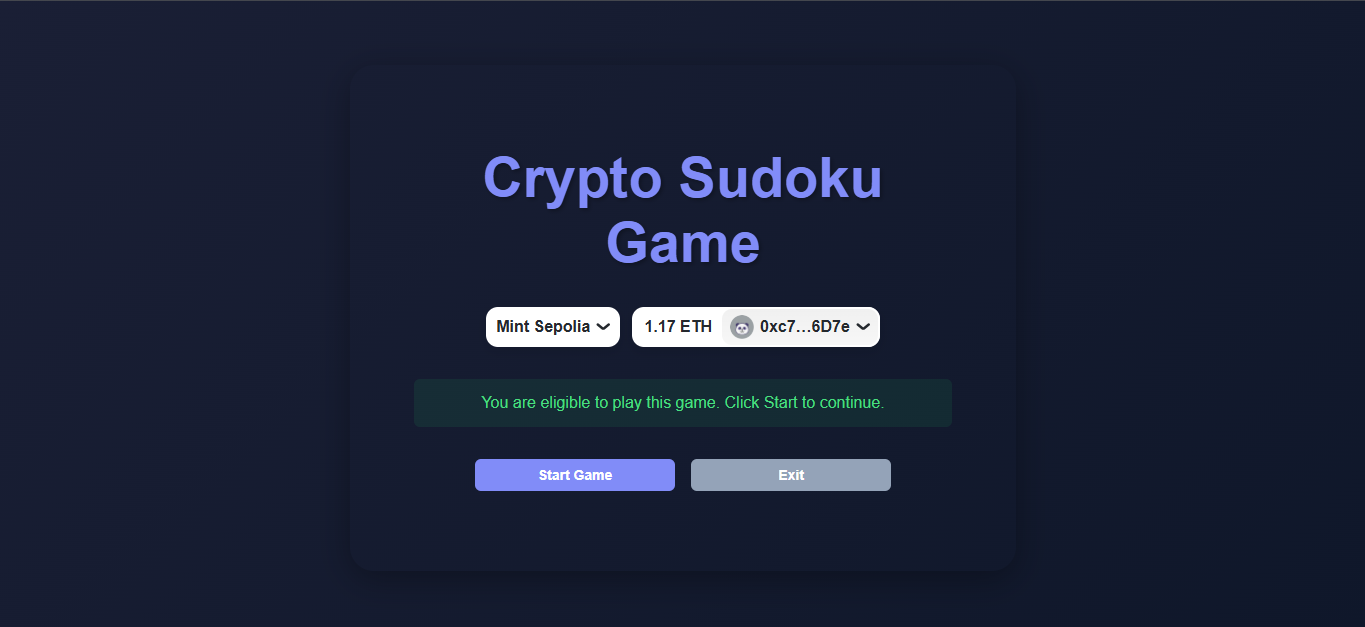
Signature
Please Confirm Signature On Your Wallet.
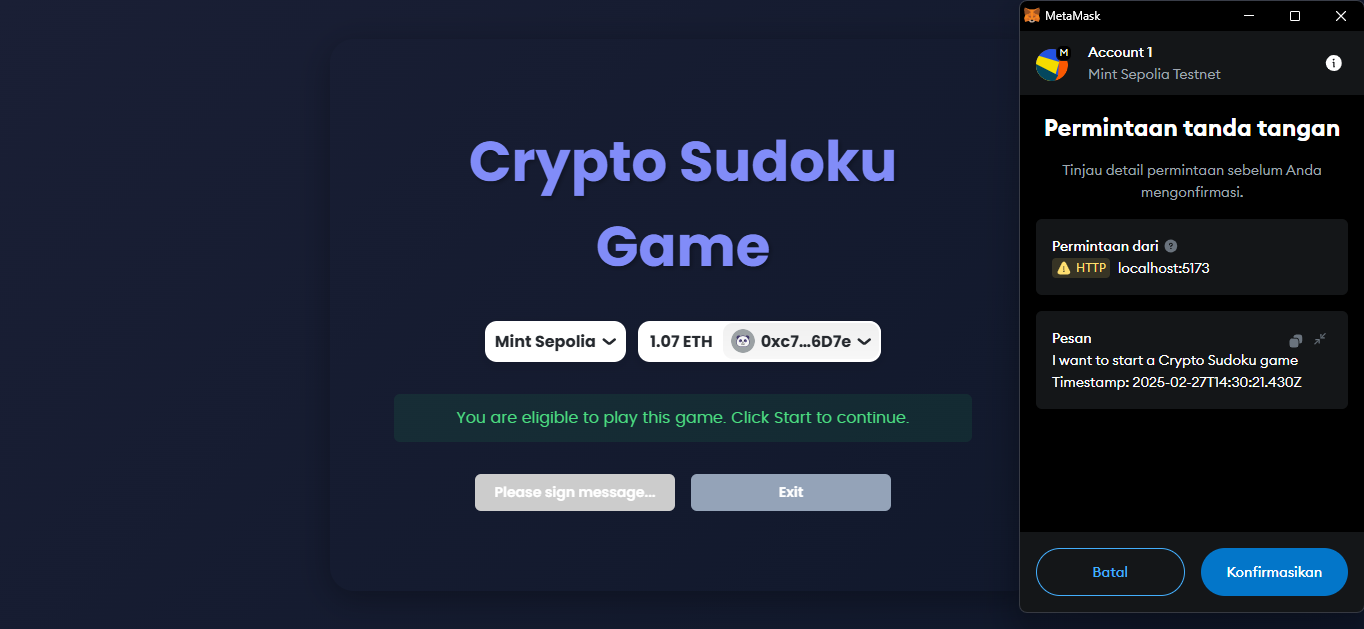
Solve the puzzle
Complete the puzzle as quickly as possible.
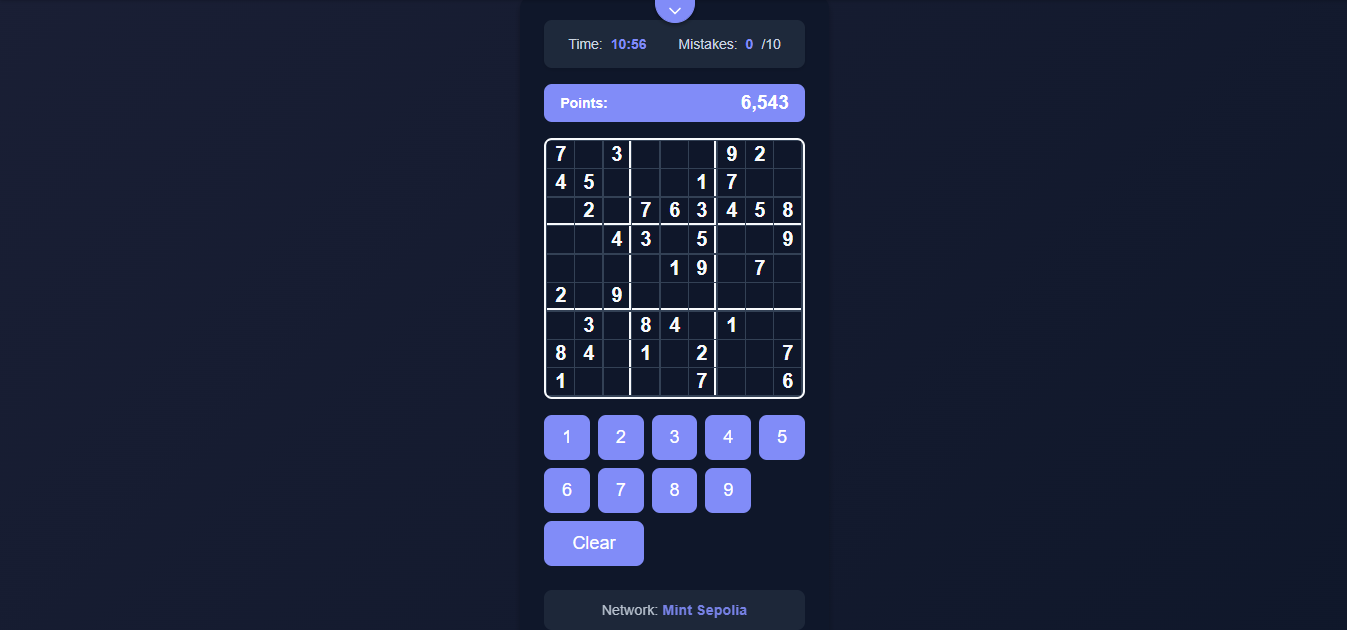
Submit Your Score
When you complete a puzzle, submit your score to appear on the leaderboard for your selected network.
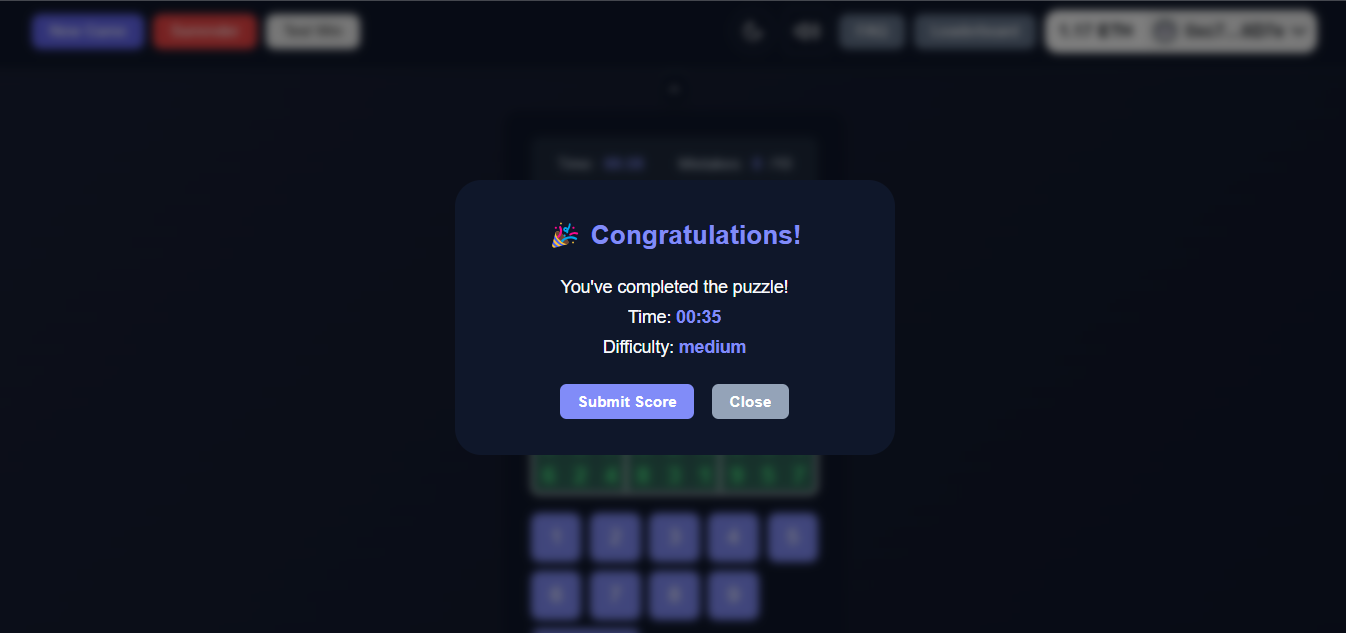
Sudoku Rules
- Fill the 9×9 grid with numbers 1-9
- Each row must contain all numbers from 1-9 without repetition
- Each column must contain all numbers from 1-9 without repetition
- Each 3×3 box must contain all numbers from 1-9 without repetition
- You have a maximum of 10 mistakes before game over
Blockchain Integration
Supported Networks
Crypto Sudoku now supports multiple blockchain networks, giving players more options to participate:
Mint Sepolia Testnet
- Network: Mint Sepolia Testnet
- Chain ID: 1687
- RPC URL: https://sepolia-testnet-rpc.mintchain.io
- Explorer: https://sepolia-testnet-explorer.mintchain.io
Monad Testnet
- Network: Monad Testnet
- Chain ID: 10143
- RPC URL: https://testnet-rpc.monad.xyz
- Explorer: https://monad-testnet.socialscan.io
Smart Contracts
NFT Contract (Mint Sepolia)
0x5E5b7277FFD01CC442184a1c2d375F421f3a1562
This contract manages the NFTs that grant access to the game on Mint Sepolia network. Limited to 10,000 total NFTs with one mint per wallet.
Leaderboard Contract (Mint Sepolia)
0x6b3fddfccfc1f7ccf54f890766e24c5d65697898
This contract stores player scores and manages the seasonal leaderboard system on Mint Sepolia.
NFT Contract (Monad Testnet)
0x74ffe581f893a630db0094757eb8f9c47108606b
This contract manages the NFTs that grant access to the game on Monad Testnet. Limited to 10,000 total NFTs with one mint per wallet.
Leaderboard Contract (Monad Testnet)
0x2a2f9179b137a1fb718f3290cb5bda730c89dec6
This contract stores player scores and manages the seasonal leaderboard system on Monad Testnet.
Score Submission Flow
Multichain Advantages
Network Flexibility
Choose the network that works best for you based on gas fees, transaction speed, or personal preference.
Increased Reliability
If one network experiences issues, you can switch to another network and continue playing without interruption.
Multiple Leaderboards
Compete in different network communities with separate leaderboard rankings.
NFT Smart Contract
NFT Access System
The Crypto Sudoku NFT contract is an enhanced ERC721 implementation with added features:
contract SudokuNFT is ERC721Enumerable, Ownable, ReentrancyGuard {
using Strings for uint256;
// Constants
uint256 public constant MINT_PRICE = 0.01 ether;
uint256 public constant MAX_SUPPLY = 10000;
// State variables
uint256 public currentTokenId;
bool public mintingActive = true;
mapping(address => bool) public hasMinted;
// Events
event SudokuNFTMinted(address indexed minter, uint256 indexed tokenId);
event MintingStatusChanged(bool newStatus);
event Withdrawal(address indexed to, uint256 amount);
}
Key features of the NFT contract include:
- Supply Cap: Maximum of 10,000 NFTs can be minted
- One-per-wallet: Each wallet can mint only one NFT (except for the contract owner)
- Enhanced Security: ReentrancyGuard protects against reentrant attacks
- Enumerable: Extended ERC721 with enumeration for better token management
- Controlled Minting: Minting can be enabled/disabled by the contract owner
- Secure Withdrawals: Uses the secure Check-Effects-Interactions pattern
NFT Minting Process
function mint() external payable nonReentrant mintingAllowed {
// Check payment
if (msg.value < MINT_PRICE) revert InsufficientPayment();
// Check if already minted (except for owner)
if (msg.sender != owner() && hasMinted[msg.sender]) revert AlreadyMinted();
// Effect: increment token ID and mark wallet as minted
uint256 newTokenId = currentTokenId + 1;
currentTokenId = newTokenId;
if (msg.sender != owner()) {
hasMinted[msg.sender] = true;
}
// Interaction: mint the token
_safeMint(msg.sender, newTokenId);
// Emit event
emit SudokuNFTMinted(msg.sender, newTokenId);
// Check if max supply reached after minting
if (newTokenId >= MAX_SUPPLY) {
mintingActive = false;
emit MintingStatusChanged(false);
}
}
The minting process includes:
- Non-reentrant function to prevent attacks
- Payment verification (0.01 ETH required)
- One mint per wallet check
- Follow the Check-Effects-Interactions pattern for security
- Automatic deactivation of minting when MAX_SUPPLY is reached
Leaderboard Smart Contract
Score Structure
Each player's score is stored in the Sudoku_Leaderboard_Optimized contract using the following structure:
struct Score {
address player; // Player's wallet address
uint32 time; // Completion time in seconds
uint8 mistakes; // Number of mistakes (0-9 maximum)
uint32 points; // Calculated points
uint32 timestamp; // When the score was submitted
}
Season Structure
Scores are organized into seasonal leaderboards:
struct SeasonInfo {
uint32 startTime; // When the season started
uint32 endTime; // When the season ends
bool isActive; // Whether the season is active
uint16 scoreCount; // Number of scores in the season
mapping(uint16 => Score) scores; // All scores in the season
}
Score Calculation
Points are calculated based on completion time and number of mistakes:
// Calculation constants
uint16 public constant MAX_TIME = 7200; // 2 hours in seconds
uint8 public constant MAX_MISTAKES = 9; // Maximum of 9 mistakes
uint16 public constant MISTAKE_PENALTY = 100; // Each mistake costs 100 points
// Points calculation formula
function calculatePoints(uint32 _timeSeconds, uint8 _mistakes) public pure returns (uint32) {
// Time points: time saved compared to maximum allowed time
uint32 timePoints = MAX_TIME - _timeSeconds;
// Penalty for mistakes
uint32 mistakePenalty = uint32(_mistakes) * MISTAKE_PENALTY;
// If penalties exceed time points, score is 0
if (mistakePenalty >= timePoints) {
return 0;
}
// Final score calculation
return timePoints - mistakePenalty;
}
Season Management
Seasons are managed through a combination of automatic and manual controls:
Automatic Season Reset
function checkAndUpdateSeason() public {
SeasonInfo storage season = seasons[currentSeason];
if (block.timestamp >= season.endTime + GRACE_PERIOD && season.isActive) {
season.isActive = false;
emit SeasonEnded(currentSeason, uint32(block.timestamp));
_startNewSeason();
}
}
Seasons automatically reset when:
- The current season's end time plus grace period has passed
- The
checkAndUpdateSeason()
function is called (either by a user submission or by the automation contract)
Manual Season Reset
function forceEndCurrentSeason() external onlyOwner {
SeasonInfo storage season = seasons[currentSeason];
require(season.isActive, "Season already ended");
season.isActive = false;
season.endTime = uint32(block.timestamp);
emit SeasonEnded(currentSeason, uint32(block.timestamp));
_startNewSeason();
}
The contract owner can manually end the current season and start a new one using the forceEndCurrentSeason()
function. This might be done for:
- Special events or promotions
- Addressing issues with the current season
- Aligning season timelines with other game events
Automation Support
function automatedSeasonCheck() external onlyAutomation {
checkAndUpdateSeason();
}
The contract also supports automated season checks through an automation contract (like Chainlink Automation), which can be configured to regularly call the automatedSeasonCheck()
function to ensure seasons rotate on schedule even if there are no player interactions.
Season Parameters
- Duration: Each season lasts 30 days (SEASON_DURATION)
- Grace Period: 1 day after end time before automatic reset
- Max Scores: Each season stores up to 100 top scores
Key Leaderboard Features
- Seasonal Rotation: Leaderboards reset every 30 days to create fresh competition
- NFT Authentication: Only NFT holders can submit scores
- Anti-Cheating: Score submissions require server-side signature verification
- Top 100 Ranking: Each season maintains the top 100 scores based on points
- Optimized Storage: Gas-efficient data types and storage patterns
Score Submission Requirements
- Player must own at least one Crypto Sudoku NFT
- Time must be within the maximum allowed time (2 hours)
- Mistakes must be 9 or fewer
- Each signature can only be used once (prevents replay attacks)
- Current season must be active
- Score must be high enough to rank in top 100 (if season already has 100 scores)